D3 Art Fun
If you came into the end product of this "drawing", you may think this is very difficult to draw. But once you recognize the pattern, it's really quite simple.With D3's path capabilities, we can draw this.
WARNING! IF YOU ARE ON MOBILE THE CURRENT D3 EXAMPLES BELOW WILL BE MISFORMATTED. YOU CAN STILL DO THEM BUT MUST ZOOM IN/OUT
Using D3 for Patterns
I am not a D3 expert, but I think I'd like to be. With D3 you can interact with data and patterns (specifically graphical representations of data, but what is a graphical representation if it isn't a pattern?). Suppose you want a timeline waterfall chart that displays projects as elements with their length representative of how many days the project is expected to last. Also, what if clicking on a project would pull up a modal form that would allow you to update information? You could do this by combining D3/CSS/JS.
If you copy this below to an HTML file, you can toy around with this sample timeline code. I will be reworking this in a future article as it's something I will actually need to use. But here you go:
<!doctype html>
<html>
<head>
<title>D3 POC test</title>
<script src="https://d3js.org/d3.v6.js"></script>
</head>
<body>
<style>
.tick > text {
transform: rotate(90deg) translate(30px,-14px);
}
#daymodal{
display: none; /* Hidden by default */
position: fixed; /* Stay in place */
z-index: 3; /* Sit on top */
padding-top: 100px; /* Location of the box */
left: 0;
top: 0;
width: 100%; /* Full width */
height: 100%; /* Full height */
overflow: auto; /* Enable scroll if needed */
background-color: rgb(0,0,0); /* Fallback color */
background-color: rgba(0,0,0,0.4); /* Black w/ opacity */
}
#dayform{
background-color: #fefefe;
margin: auto;
padding: 20px;
border: 1px solid #888;
width: 90%;
}
</style>
<div id="viz" style="width:2000px;height:500px;border:1px lightgray solid;">
</div>
<div id="daymodal">
<div id="dayform">
Enter the length of days for this project:
<input type="text" id="ldays">
<input type="submit" id="submit" value="Submit">
</div>
</div>
</body>
</html>
<script>
// Set Dimensions
const xSize = 2000;
const ySize = 500;
const margin = 70;
const xMax = xSize - margin*2;
const yMax = ySize - margin*2;
// Create Random Points
const numPoints = 25;
const dates = [];
for (let i = 0; i < numPoints; i++) {
var date1 = randomDate(new Date(2022,0,1), new Date(2025,11,31));
var date2 = randomDate(date1,new Date(2025,11,31));
var name = "project"+i;
dates.push([date1, date2, name]);
}
dates.sort(function(a,b){ return a[0]-b[0] });
const svg = d3.select("#viz").append("svg")
.attr("width", xSize).attr("height", ySize)
.attr("font-family", "sans-serif")
.attr("font-size", 10)
.style("display", "block")
.append("g").attr("transform","translate("+margin+","+margin+")");
const yearsList = [];
const maxDate = d3.max(dates, function(d) { return d[1]});
const minDate = d3.min(dates, function(d) {return d[0]});
const numberOfMonths = Math.ceil((maxDate-minDate)/(24*3600*1000*(365/12)));
const numberOfDays = Math.ceil((maxDate-minDate)/(24*3600*1000));
const projCount = dates.length;
for (let i=0; i<numberOfMonths; i++){
let tempYear = minDate.getYear()+1900;
let tempMonth = minDate.getMonth();
let numYears = Math.trunc((tempMonth+i)/12);
let calcMonth = (tempMonth+1+i) % 12;
if (calcMonth == 0){
calcMonth = 12;
}
yearsList.push((tempYear+numYears)+"-"+ calcMonth);
}
const x = d3.scaleBand()
.domain(yearsList)
.range([0, xMax]);
svg.append("g").attr("transform", "translate(0," + yMax + ")")
.call(d3.axisBottom(x));
svg.append('g')
.selectAll("projs")
.data(dates)
.enter()
.append("rect")
.attr("x", function (d) { return calcX(d) } )
.attr("y", function (d,i) {return calcY(i)})
.attr("height",function() {return Math.floor(yMax/(projCount)) })
.attr("width",function(d) {return calcWidth(d)});
svg.append('g')
.selectAll("projs")
.data(dates)
.enter()
.append("text")
.text(function (d) { return d[2]})
.attr("fill","red")
.attr("x", function (d) { return calcX(d) } )
.attr("y", function (d,i) {return calcY(i+1)});
function randomDate(start, end){
return new Date(start.getTime() + Math.random()*(end.getTime() - start.getTime()));
}
function calcX(project){
var numbFromBeg = (project[0]-minDate)/(24*3600*1000);
return (numbFromBeg/numberOfDays*xMax);
}
function calcY(index){
return (index * (yMax - (yMax/projCount))/projCount);
}
function calcWidth(project){
var projDays = (project[1]-project[0])/(24*3600*1000);
return projDays/numberOfDays*xMax;
}
document.addEventListener('click', function (event){
var clicked = event.target;
if(clicked.tagName == 'rect'){
document.querySelector("#daymodal").style.display = "block";
document.querySelector("#submit").onclick = function (){
var nmdays = document.querySelector("#ldays").value;
clicked.setAttribute("width", nmdays/numberOfDays*xMax );
document.querySelector("#daymodal").style.display = "none";
};
}
});
</script>
Here is a little demonstration that kinda works in this embedded context:
What's the pattern here? In this case it's our collection of ordered projects (ordered by when they start) and displayed in a reliable way (width calculated by the number of days a project will last). D3 gives us a quick way to inject data into a scaffold where we can control how it's displayed (we get to decide the pattern). The rest of this article will show another pattern we can use D3 to demonstrate.
Fun Art Pattern
There's a fun way to make an interesting square spiral drawing by hand. It's hard to lightly put the pattern in words, but start with a square or rectangle. Pick a starting corner to draw from. Going clockwise (or counter clockwise as seen below), draw from this corner to a point approximately 1/8th the side between the next corner and the diagonal corner from where you started (this would be on the opposite side from the starting point). Now, from this new point, select a point about 1/8th the length of the side opposite your current position and draw a straight line to this next point.
Continue repeating this process until you are tired of the exercise and you can end up with a cool looking spiral square tunnel like thing. Here is a demonstration I created in paint:
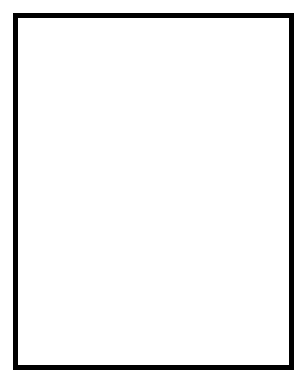
If you came into the end product of this "drawing", you may think this is very difficult to draw. But once you recognize the pattern, it's really quite simple.With D3's path capabilities, we can draw this (note that D3 is not the only way to work with paths, but D3 will allow us to easily work with a collection of data to build the collection of paths that make the pattern). We really have 2 major concerns here. First, we will need a way to generate the points of our lines. Second, we will need a way to render those lines. The first part is the hardest, but if we make it so that we can get a collection of drawing points then the second part will be quite easy (we just have to connect the points).
Calculating the Paths
There are several ways we could decide to build the path points. As stated, the above example works by approximately bisecting the next inner square side by 1/8th. You could also get a similar result by continually drawing a line from one point to the next based on a set angle. There are other ways you could propose to continually make smaller and smaller squares, but the more complex the generation of the next smaller square, the more complex your algorithm will need to be.
This seems to be the simplest algorithm I can think up.
- Let L be the number of path points we want to generate.
- Let z be the divisor used to subdivide the destination line and determine each "next" point.
- Let our starting rectangle have the dimensions of n*m.
- Let R be an object array of size 4 that will be used to store the 4 "current" vertices of our innermost lines. The array should be initialized to indicate the vertices of our starting rectangle in an ordered way (the order of our spiral in a clockwise or counter clockwise fashion).
- R[0] = {0,0}
- R[1] = {n,0}
- R[2] = {n,m}
- R[3] = {0,m}
- Let P be an object array of size L that will store the path coordinates we will draw lines to.
- for i=0, i<L, i++ (for non programmers, we will perform a loop L number of times and use "i" to refer to the current number of loops performed - remember a we are generating points right now and L refers to how many points we want to create)
- Set R[(i+1) MOD 4] to the point on the line segment R[(i+1) MOD 4] to R[(i+2) MOD 4] that is 1/z from R[(i+1) MOD 4]. (What we are doing here is we are using the innermost lines between our points to determine where on this line the next point is, then we are setting this as a new most inner point in our R array).
- Set P[i] to R[(i+1) MOD 4]. (And now we must also record this point in our point array so we can use D3 to draw a line to it).
- Use D3/path to render the collection P
What did you just read? Several things going on here.
What is MOD 4? This indicates we are using the modulus function to keep us rotating in our array of 4 vertices. In programming, we typically use '%' to indicate the modulus operator. Some examples: 4%4 = 0 and 4%5 = 1 and 9%4 = 1. It indicates the remainder of one integer divided by another. We are looking ahead to the next 2 vertices of our square in this loop. We do this to look at what that line segment is and determine where our next vertex should be.
The sub steps of our loop need to be flushed out a little more. How do we determine a point 1/zth away from the old vertex, R[(i+1) MOD 4]? We add (x2-x1)/z to x1 and (y2-y1)/z to y1. This is putting the concept of slope in algebra to good use. Once this point is determined, we need to update the vertices of our square and also add the new point to our path array, P.
There is one more thing of note. The above algorithm works to draw a counterclockwise pattern starting from the origin (0,0) of the bottom left corner of our drawn square IF that square were oriented in Quadrant 1 of a Cartesian plane. But SVG graphics work so that a positive y value actually moves down the screen. So with the R coordinates set in the order that they currently are, this will actually create a clockwise pattern from the top left origin of the square. If starting from the bottom left is desired, I believe you could probably reorder the coordinates of R so that R[0] is the (0,n) coordinate and shift all of the other elements of R accordingly.
Here you can view a demo of my implementation. Checkout the difference when you change the divisor from the 40 default below to a smaller number like 10.
x:
y:
Divisor of line segments (10 => 1/10th):
Number of vertices to generate (int):
Below is the sausage making function using D3.
function calculatePoints(){
document.querySelector("#modal").style.display = "block";
var n = Number(document.querySelector("#xin").value);
var m = Number(document.querySelector("#yin").value);
var z = Number(document.querySelector("#zin").value);
var L = Number(document.querySelector("#lin").value);
console.log(n);console.log(m);console.log(z);console.log(L);
var vertices = [];
vertices[0]= {x:0, y:0};
vertices[1]= {x:n, y:0};
vertices[2]= {x:n, y:m};
vertices[3]= {x:0, y:m};
const points = [];
for (let i=0; i<L; i++){
let xOffset = (vertices[(i+2)%4].x - vertices[(i+1)%4].x)/z;
let yOffset = (vertices[(i+2)%4].y - vertices[(i+1)%4].y)/z;
vertices[(i+1)%4].x = vertices[(i+1)%4].x + xOffset;
vertices[(i+1)%4].y = vertices[(i+1)%4].y + yOffset;
points.push({x: Math.floor(vertices[(i+1)%4].x), y:
Math.floor(vertices[(i+1)%4].y)});
}
console.log(points);
var path = d3.path();
path.moveTo(0,0);
for(let i=0; i<L; i++){
path.lineTo(points[i].x, points[i].y);
}
const svg = d3.select("#viz")
.append("svg")
.attr("width", n).attr("height", m)
.attr("font-family", "sans-serif")
.attr("font-size", 10)
.style("display", "block");
svg.append("g")
.append("rect").attr("width", n).attr("height", m)
.attr("stroke", "black")
.attr("fill","white");
svg.append("g")
.append("path").attr("d", path)
.attr("stroke", "black").attr("fill", "white");
}
For more fun, you could alter the code to just display the points and not draw the path.
1/14/25 edit - Added mobile warning, adjusted a few misformatted code bits, and slightly changed layout of article