Binary Representation of Integers
Beware! If we try to use numbers too big for an int we can run into unexpected results. What happens if we add 1 to 2,147,483,647?
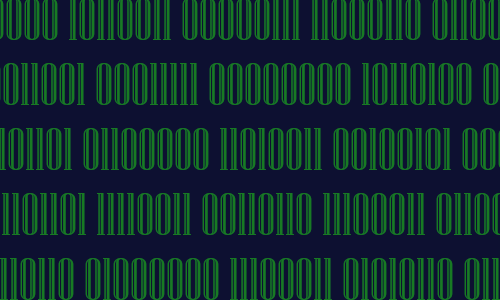
The Way a Computer "Thinks"
You may have heard "it's all just 0's and 1's." A processor works through a program by loading its list of compiled instructions that are stored as binary (or a number of base 2, or more simply, a number consisting only of 0's and 1's). A single digit of a binary number is called a "bit" (Binary digIT). When you hear of a 64 bit processor, we are talking about a processor that can handle binary numbers that are 64 bits long. For completeness, 8 bits are called a byte.
What's with binary? This is because computer logic hardware within the processor works with binary state (like an on/off light switch, but many of them, and they use 1/0 to indicate the state). Binary numbers represent instructions in the CPU's instruction set, addresses in memory, registries, interface devices, and data. Binary data stores encoded information consisting of numbers, text, images, and various media waiting to be read and possibly manipulated by a computer program.
The goal of this article is to look at how integers are stored in programs. We will be using C# to investigate, though this really isn't language specific.
The int Type
The int type in C# (and many other languages) is used to store integer (or whole or non-decimal) numbers. A value of this data type allocates a specific amount of space in memory: 4 bytes or 32 bits. The following line of code would give us a confirmation of this:
Console.WriteLine($"Size of an int: {sizeof(int)} bytes");
Size of an int: 4 bytes
Although we are working with 32 bits, one bit is used to store if the number is negative or positive. The leading bit is used for this, where a 0 indicates a positive number, and a 1 indicates a negative number. This leaves us the remaining 31 bits to store the magnitude of the number. This gives us the total magnitude scale of
231=2,147,483,647
With the leading bit, we get to spread this almost evenly across the negative number range as well. Because we don't need to represent a -0 value, we use a scheme called two's complement to convert negative values and get down to -2,147,483,648.
The way two's complement works is by taking the negative number's 31 bits and converting 0's to 1's and 1's to 0's and then adding 1 to the resulting value. So, although it seems like
10000000 00000000 00000000 00000000
should be -1, it is actually -2,147,483,648.
We can confirm this with a bit of code:
string binAllOne = "11111111111111111111111111111111";
string binLeadZero = "01111111111111111111111111111111";
string binAllZero = "00000000000000000000000000000000";
string binLeadOne = "10000000000000000000000000000000";
int allOnes = Convert.ToInt32(binAllOne, 2);
int leadZero = Convert.ToInt32(binLeadZero, 2);
int allZero = Convert.ToInt32(binAllZero, 2);
int leadOne = Convert.ToInt32(binLeadOne, 2);
Console.WriteLine($"{binAllOne} as INT: {allOnes}");
Console.WriteLine($"{binLeadZero} as INT: {leadZero}");
Console.WriteLine($"{binAllZero} as INT: {allZero}");
Console.WriteLine($"{binLeadOne} as INT: {leadOne}");
11111111111111111111111111111111 as INT: -1
01111111111111111111111111111111 as INT: 2147483647
00000000000000000000000000000000 as INT: 0
10000000000000000000000000000000 as INT: -2147483648
Effectively, the int type gives us the number range of -2,147,483,648 to 2,147,483,647.
Unexpected Results
Beware! If we try to use numbers too big for an int we can run into unexpected results. What happens if we add 1 to 2,147,483,647? When we do that we are altering the leading bit value and we wrap around from positive values to negative values. This can work both ways. Check this out:
string binLeadZero = "01111111111111111111111111111111";
string binLeadOne = "10000000000000000000000000000000";
int leadZero = Convert.ToInt32(binLeadZero, 2);
int leadOne = Convert.ToInt32(binLeadOne, 2);
Console.WriteLine($"{leadZero}+1: {leadZero + 1}");
Console.WriteLine($"{leadOne}-1: {leadOne - 1}");
2147483647+1: -2147483648
-2147483648-1: 2147483647
If we don't need to use the negative number space, we can specify that we want to use an unsigned int. This allows us to use all 32 bits to express a positive magnitude.
//unsigned int
Console.WriteLine($"Size of an unsigned int: {sizeof(uint)} bytes");
uint unsignedAllOnes = Convert.ToUInt32(binAllOne, 2);
Console.WriteLine($"{binAllOne} as Unsigned Int: {unsignedAllOnes}");
Size of an unsigned int: 4 bytes
11111111111111111111111111111111 as Unsigned Int: 4294967295
We mentioned 64 bit processors in the beginning of this article. Not too, too long ago, many processors were 32 bit. This meant that they only had 32 bits to address the bytes available. How many GigaBytes is this? A GigaByte is:
230 bytes
This means that at a byte addressable level, a 32 bit processor could address:
232/230=22 GigaBytes or 4 GigaBytes
Older readers may remember that many of their old XP machines couldn't have more than 4 GB of RAM. This was all the memory that could be addressed!
Bigger Numbers
It could be that you need an integer value still larger. There is a data type that can help (with either signed or unsigned options available). This is the long data type.
Console.WriteLine($"Size of a long: {sizeof(long)} bytes");
Size of a long: 8 bytes
This is more than enough to handle most real world needs. 8 bytes utilizes all 64 bits of a processor.